Everything you need to know to get started with Rust
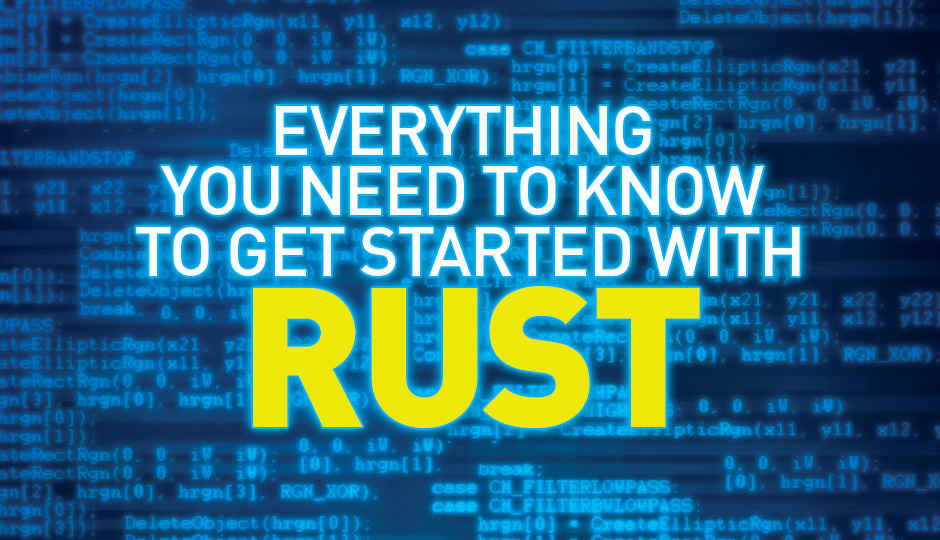
Rust is very much in development, but if things go well it will have a bright future.
Rust has an interesting history, especially since it is very much still in the making. Seeing the limitations of browser engines that started development back in the days when text was the main component of web pages, Mozilla began development of Servo, a next-generation web engine that would be highly parallelised. It would take advantage of the growing trend of multi-core CPUs on devices, and modernising the way web content is rendered. Current generation language such as C and C++ also began to show their age and inadequacy when faced with the task of coding such an engine. Rust was the answer to a problem Mozilla created for itself; where will the web be in another decade? Just as Rust is currently in constant development, so is its most major project, Servo. A complex project like a web rendering engine is the perfect testbed for a language. Ten years from now, Servo might just be what is behind Firefox — or Mozilla’s next browser — and Rust will be what is behind Servo.
Rust claims to have a focus on “high-level, bare-metal programming” and this is quite evident once you start exploring the language. First of all the official implementation of Rust is a compiler that produces a binary file. Secondly it includes many features found in languages like Python and Ruby that greatly improve developer productivity. Despite this it also offers many of the power features of low-level languages like C, such as pointers. In fact since it has started development at a time when multi-core computers are so popular, it also makes it simpler than ever to write multi-threaded applications.
Servo is already passing the ACID2 test, and has remarkably better performance than current the Firefox Gecko engine.
One of the big goals of Rust is to be a safe language despite being low-level; to not let performance compromise safety. This has lead to some interesting design choices for the language. Rust takes many cues from functional programming languages, and has many features — such as patten matching — that will be familiar to those who like functional languages. In fact variables in Rust are immutable (meaning they can’t be modified after assignment) by default. While Rust was started by Graydon Hoare as a personal project at Mozilla at this point Rust is a community driven project. This is the perfect opportunity then, to get onto Rust on the ground floor, and not only learn a language that might be significant some day, but to shape it.
Significance
Rust is a language that is still very much in development, so its future is hard to evaluate right now. Given that it has been around for 5 years, is still going strong, and that it has a large organisation like Mozilla behind it, it won’t simply disappear off the face of the Earth suddenly one day. It is also a language strongly tied to the development of Mozilla’s future browser, so it has at least one major software baked in. The syntax and semantics of Rust are still in flux. This can certainly be a negative for those trying to learn a language. Especially to find that the perfectly good program run one day and fail in the very next release. However, for those interested in studying programming languages, and their development, this is a great opportunity.
While Go is new, and publicly developed with community contributions, Rust is in an earlier and more exciting phase where there is more scope for change, and backward compatibility isn’t much of an issue. For someone who wants to be involved in the development of a programming language, this is a perfect opportunity. Even if you not interested in this aspect of programming, seeing the development of a language can give you insight into the programming language you currently use. Even before releasing a first stable version, or reaching a stable specification, it has begun to influence other languages. For instance it was one of the language that influenced Swift, Apple’s recently released programming language for iOS and OSX development.
The above simple code sample runs each iteration of the loop in a separate thread. Each time you run this code, the order of the output will be different depending on the order in which the threads finish executing.
The most interesting thing about Rust is that it is incredibly simple to do seemingly complex things. For example creating concurrent applications that run multiple threads where each works on bits of the same problem while coordinating with a main thread; this can be a pain in many languages. However solving this challenge is something that Rust is built around and as such you might find the syntax for doing such things simpler than in many other languages. For example if you wanted to simply create an application that prints “Hello World” from a separate thread, it would be as simple as: ‘spawn(move || {println!(“Hello, world! {}”, x);});’ Note that the above syntax may change (it already has from the stable 0.12 to 0.13 in development).
Rust also has an intricate system for controlling access to pointers such that you never end up in a situation that causes crashes due to incorrect use of pointer. It’s also better at tracking pointer memory usage without major performance penalties.
Finally, Rust also makes it easy to integrate with legacy C code with efficient C bindings.
Hello World
fn main() {
println
!(“Hello, World!”);
}
Will this still work as a Hello World app in a year, we don’t know — it most probably will — but it sure does right now! Rust keeps things simple when you’re trying to do simple things while still having enough power to accomplish complex things when needed.
Sort
fn insertion_sort(
uarr
: &mut [int]) {
for idx in range(1u, uarr.len()) {
let curr_value = uarr[idx];
let mut pos = idx;
while pos > 0 && uarr[pos - 1] > curr_value {
uarr[pos] = uarr[pos - 1];
pos -= 1;
}
uarr[pos] = curr_value;
}
}
On a glance this might look like just some C or C++ code, but there are some interesting differences. For one, there are no brackets around the expressions following for and while. Another noticeable difference is that you do not need to declare the type of a variable if that is obvious from the declaration.
You will also notice that we declare ‘curr_value’ as ‘let curr_value = uarr[idx];’ while for ‘pos’ we declare ‘let mut pos = idx;’. This is because by default variables in Rust cannot be changed once set; so if we want a variable that need to be constantly modified, we need to explicitly declare it as a mutable variable. Since we want to modify the incoming array to sort it, the array needs to be mutable as well.
Tools and Learning Resources
Given that Rust is still heavily in development, and the syntax of today might not work tomorrow, the best place to learn the language is the official website. While other tutorials and blog posts that introduce the language might exist, they are likely to be out of date by the time you read them. The official documentation and guides that are offered on rust-lang.org are kept up to date as the language evolves, so you can be sure that the information there is correct, and up to date. It also helps that the official documentation of the language on its website is very good and detailed. Rust is also so early in development currently that there are no IDEs that officially support it. The closest you will get are plugins for Eclipse and JetBrains’ IDEA IDEs that add support for the Rust language. The IDEA plugin can be installed from the the IDE’s plugin management system, and can be found by searching for Rust. Currently this plugin does not support anything other than syntax highlighting for Rust source code, although that is planned and coming soon. The Eclipse plugin is called RustyCage and offers a few more features.
Atom, Sublime Text and Emacs also have plugins available that add syntax highlighting and autocompletion support for Rust. For Vim lovers there is a a syntax highlighting plugin available. Currently the best support for Rust is available for Sublime Text. Its plugins allow for not only syntax highlighting and autocompletion, but also compiling and running applications from within the editor. If you’re looking to download the Rust compiler itself, you’ll find that it’s currently a bit harder than installing most other languages due to the language still being in development. The only Linux repositories where you will find the rust compiler are unofficial ones, that may or may not keep it updated.
A syntax highlighting plugin can be easily installed for GitHub’s free Atom code editor.
The best way to get the rust compiler and libraries then is through the official website that offers 32bit and 64bit bit packages for Linux, OSX and Windows. The website also offers two versions of the language, a ‘stable’ version (currently at 0.12) and a nightly version that is constantly updated. The recommended version is the nightly version since the syntax of the ‘stable’ version is usually already outdated with the continual development of the nightly version.
For tutorials on 15 other hot programming languages go here.