Everything you need to know to get started with Ruby
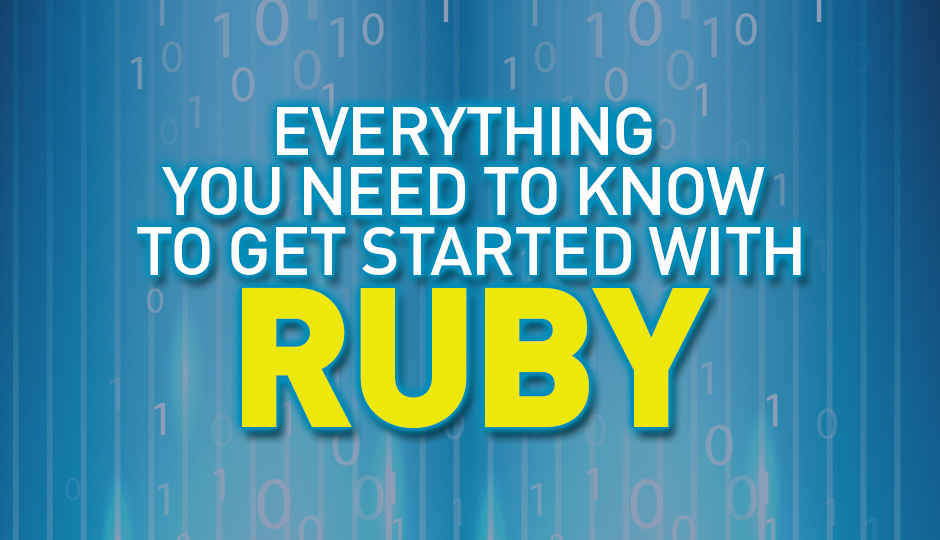
Ruby is a language that takes the term object-oriented very seriously and was built from the ground up to be object-oriented.
This isn’t a slight against the language though! It has continued to evolve in the duration, with the release of 1.x versions. In fact Ruby 1.9, which released in 2007, changed the language in incompatible ways, such that many scripts that worked with 1.8 needed to be rewritten to support it. On the other hand the more major-sounding release, Ruby 2.0 was largely compatible with previous versions, and the same can be said of 2.1 — both released in 2013.
Ruby is the creation of Yukihiro Matsumoto, who felt that there was a lack of proper object-oriented languages, and that on most languages it felt like the feature was tacked on. Ruby was his attempt at such a language. The result of that decision is that everything is an object in Ruby. While some languages differentiate between ‘raw’ data types such as integers and floats, and more complex object-oriented datatypes it supports, that is not the case with Ruby. Even integers and floats are objects which allows for odd looking things such as calling methods on integer or float values (3.1412.to_s() for instance will convert the number 3.1412 to a string).
Yukihiro Matsumoto, the creator of Ruby hard at work on Ruby, we assume.
Another aspect of everything being an object in Ruby, is that even your own code is an object that can be manipulated. In other words, Ruby supports meta-programming. Even classes and functions are objects in Ruby, and can as such be created, modified, passed around and used as return values in Ruby.
Ruby even has ‘open’ classes, so a class created elsewhere, by someone else, in a different module can be edited by you in your own code to add features. This is not the same as subclassing, which is also supported, you are modifying the class itself. You can even modify core classes, such as the inbuilt Integer class to add features to integer numbers. For instance you could add an ‘is_prime’ method to the Integer class, and then be able to quickly check if any number is prime as 17.is_prime or 9.is_prime .
Given what we’ve just said about how easy Ruby makes it to modify things, it might seem odd that Ruby also supports functional programming. Ruby goes through the trouble of marking any method that has side-effects. Any method on an object that changes the internal state of that object is marked with a ‘!’ at the end. So if you have an array called ‘arr’, calling ‘arr.sort’ will return a sorted version of the array, while ‘arr.sort!’ will sort the array itself in place. This allows people who wish to program in a functional paradigm to do so. Where Python takes the view that it is best to provide one way to do things (i.e. not have many alternate forms of syntax for the same thing) Ruby takes the opposite view, that having different ways of doing the same thing is good. Sometimes doing things a different way makes them clearer if not better. Which view is better? We say that’s a silly question, and its silly to rank subjective views on an objective scale.
Significance
If we had one word to explain the significance of Ruby, that word would be ‘Rails’. Ever since the release of ‘Ruby on Rails’ in 2005, the framework has taken the world of web development by storm. Like it or hate it, it’s impossible to deny that it has had a huge impact. In fact it was initially the framework of choice of Twitter — they have since moved to other technologies — and today it runs popular websites such as GitHub and Hulu. Rails is a framework for creating websites that is built on Ruby, that embraces and encourages many software engineering best practises. It greatly simplifies building a RESTful website by automating a large part of the process using auto-code-generation tools and a philosophy of “convention over configuration”. Ruby is also the language of choice of the open-source social network software diaspora*. While the project never reached critical mass — or it hasn’t yet — the choice of technology for such a project is critical. The diaspora* is not only open-source, but also decentralised, allowing people hosting their profile on different servers to be friends.
The diasopra* social network introduced features like contact groups (circles) that are now available in both Google+ and Facebook.
Another major consumer of Ruby is YaST (Yet another Setup Tool), a collection of tools for Linux administrators to configure different parts of the system. It is software developed by the OpenSUSE community for their distro. YaST was originally written in a custom programming language called YCP, which had the obvious disadvantage that only developers of YaST knew it or cared about it. For their 13.1 release in 2013 that developers decided to switch to Ruby due to its popularity with developers and the chance to inject fresh blood to this important project. Ruby, while fluctuating up and down on the TIOBE rankings, still happens to stay in the top 20 languages. It ranks highly (4th) among the languages on GitHub though, with over a hundred thousand active repositories.
Perhaps the most popular and significant thing to come with Ruby though is Rails. Its flexibility and ease of use, along with easy deployment to hosts such as Heroku, has given it a huge boost and put it in great demand.
It’s a simple language to learn, yet quite powerful. It has also has the advantage of a large community, and thousands of great libraries and packages.
The Software Installer component of YaST is a powerful way to search, filter and install applications on OpenSUSE Linux.
Hello World
puts “Hello, World”
Ruby is yet another one of those languages that doesn’t need large amounts of boilerplate just to push basic things like a “Hello, World” message to screen.
Sort
def insertion_sort(
uarr
)
1.upto(uarr.length - 1) do |idx|
curr_value = uarr[idx]
pos = idx
while pos > 0 and uarr[pos - 1] > curr_value
uarr[pos] = uarr[pos - 1]
pos -= 1
end
uarr[pos] = curr_value
end
end
This little piece of code might not be the best representation of the Ruby approach to problem solving.
A similar ‘real’ example would embrace Ruby features like open classes by adding the feature directly to Ruby’s Array class. Also since this function is trying to modify the array, and as such has side effects it should end with a ‘!’.
That said, this code does show some of the elegance of Ruby, such as the use of constructs like num1.upto(num2) for iteration. Ruby has many such idioms that make programming pleasant.
Tools and Learning Resources
Given the vast community Ruby enjoys, there is no shortage of tutorials dedicated to learning the language. Ruby happens to have a website dedicated to offering detailed interactive tutorials that are not only brilliant, but also free. You can find them at rubymonk.com
Another great website that tests you as you go along is rubykoans.com. However this one is best experienced after you have at least the basic fundamentals of some programming language down. We feel it works better when introducing you to Ruby as a second language rather than the first.
One of the most unconventional (and as such most interesting) tutorials to the language you will find is called “Why’s (Poignant) Guide to Ruby”. If you don’t want to read it for Ruby, read it for the cute illustrations of anthropomorphised foxes. Also it’s the only programming language tutorial / guide we can think of that comes with its own soundtrack. You can find this book here
A great deal of effort and love seems to have been put into “Why’s (Poignant) Guide to Ruby” to try and make something as dull as learning a new programming language entertaining. The fact that it’s free and under Creative Commons is ever more heartening.
So now that know where to go if you want to get started with Ruby, you probably want to get it installed on your computer. If you are using a Linux or Unix distro, you’ll be happy to know that your distro’s repositories will most definitely have it available. For Windows and OSX users, the best place to start is ruby-lang.org the official website of the Ruby programming language. For Windows users the best version to get is probably the one available at railsinstaller.org since it also includes Rails (which you’ll probably want to check out) and also a number of additional Ruby third-party components. If you just want to dive into Rails, and want a full stack including a web server and database server check out Bitnami’s Ruby stack. A must-have tool if you want to do serious work with Ruby is RVM (Ruby Version Manager). This brilliant package lets you use multiple Ruby versions, and multiple Ruby package versions without getting into a confusing mess. You are likely to find this very useful if you have multiple projects using different versions of the same packages. Installing RVM on Windows is possible, but not directly, it will only work under Cygwin, a Linux compatibility layer for Windows.
Bitnami’s stack is great if you are getting into Ruby for web development, since it includes web and database servers, and a simple tool to manage them.
Like most programming languages that have been around for a while, Ruby too has a number of alternative implementations. These can be more appropriate than the official implementation based on what you need to do with Ruby. JRuby for instance runs on the JVM and gives you access to Java classes. IronRuby runs on .NET and lets you use it’s features. Rubinius is another alternative implementation of Ruby that uses a JIT compiler for better performance. If you’re looking to develop for iOS and Android, RubyMotion is the implementation to check out, although it is not free like the ones mentioned above. Also unlike the above, it compiles your Ruby code into an app for those platforms — which it would have to for iOS to not run afoul of its rules. If you like Ruby and are looking for a cross-platform way to build a mobile app, it might be worth the money. One last tool we’d like to mention, is Pry, which is an alternative to the default Ruby shell (irb). The default Ruby shell is — to put it kindly — functional, Pry greatly improves on the default shell, and it is just a single command away (`gem install pry`).
The Pry shell, unlike the default shell irb, supports syntax highlighting and code auto-completion.
For tutorials on 15 other hot programming languages go here.