Everything you need to know to get started with Python
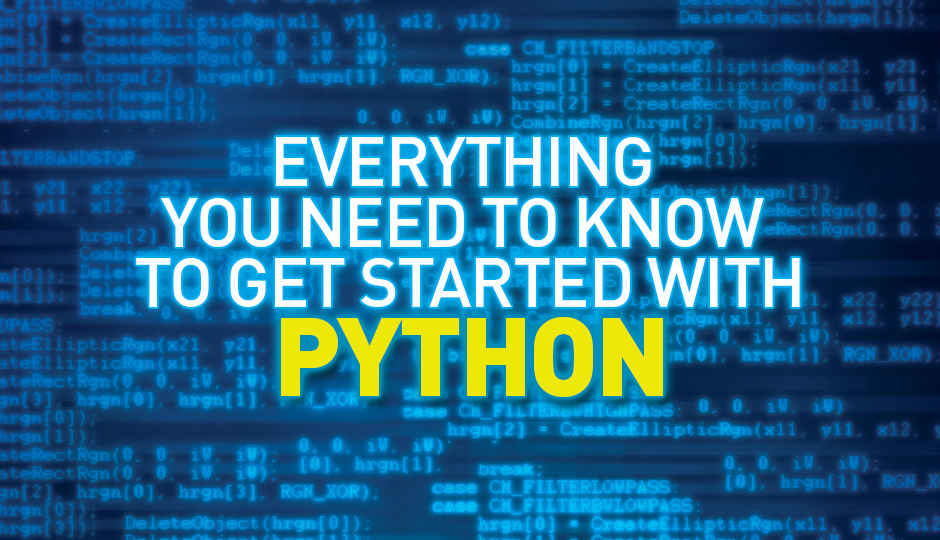
Python is probably one of the best languages to start with if you are new to programming and it continues to be useful later as well
Python might seem like a recent development but at this point it is 20 years old. The first release of Python 1.0 goes as far back 1994. Even the Python 2 series which is the dominant version of Python to this day was released way back in 2000. The language was created by Guido van Rossom and he continues to have a central role in its development. Python is in a rather odd situation currently where the most popular version of the language is the Python 2 variant despite the availability of Python 3 since 2008. Python 2 had its final major release in 2010 with the release of Python 2.7 and it will only be bug fixes from here. Python 3 itself is currently at 3.4 with 3.5 to release next year.
Guido van Rossum, the creator of Python worked for Google while they launched Google App Engine with Python support out of the box. He now works for Dropbox.
This preference for Python 2 comes from the fact that the Python 3 breaks compatibility with Python 2 code. Python 3 was a major update to the programming language and the developers took the opportunity to modernise the language in many ways and get rid of poor decisions made in the past.
The syntax differences between Python 2 and 3 kept a majority of Python libraries from instantly supporting it, and some are yet to support it. While there is an automated tool for converting Python 2 code to Python 3 code, it doesn’t work in all cases. The tides are slowly changing as Python 3 gains new and enticing features, and more libraries are ported to it. To further ease this process Python 3.3 brought its syntax closer to 2 and made it easy to make code compatible with both versions. While this might make it sound like they are very different languages, they are really not, it’s just the nature of the changes that has caused these issues rather than their intensity or quantity.
With talk of versions out of the way, let’s look at the language itself, which is unconventional in many ways. Python takes a very different approach to structuring code, rather that denote blocks of code with curly braces {}, it uses whitespace indentation. Most languages keep the formatting of the code separate from syntax, which means you are likely to run into dozens of different coding styles and arguments over which is better. Python makes the indentation part of the language resulting in more consistent looking code.
Python has a core philosophy, and the more you code in Python the more you tend to understand what makes certain code more or less ‘Pythonic’. Long time Python developers can be said to have gotten the ‘The Zen of Python’. You can read ‘The Zen of Python’ on their website, or by typing `import this` in a Python console.
Significance
Possibly one of Python’s greatest strengths is its simplicity. It is easy to get into it. Python is widely used, to the point that it is present by default if you’re running OSX. The same can be said of most Linux distributions. Installing Python on Windows isn’t that hard either.
When you reach the limits of what can be accomplished using the Python’s inbuilt libraries, there is an extensive ecosystem of packages out there to explore. The PyPI or Python Package Index is a website that lists all Python packages submitted to it. This index of packages is accessible from the command line with the `easy_install` and `pip` which lets you search this index, download packages and update them if need be. You can just list all the packages you want for you project in a text file and `pip` can install them all automatically. Python packages are diverse, with libraries that integrate with APIs, GUI programming frameworks, mathematical and biological computation, web frameworks and more.
If your designs are more mercenary, you’ll be glad to know that there is healthy demand for Python programmers, and it has been placed at #8 or above in the TIOBE Programming Community Index from many years. It is used by major organisations like Google — where its creator worked till 2012 — and scientific research organisations such as CERN. A popular video hosting website called YouTube is also built on Python, and it is used behind the scenes on other Google products as well. DropBox also uses Python, and in fact currently employs Guido. Another high-traffic website, Reddit is built on Python and is in fact open source. The popular Discus commenting platform is also built on Python, using the Django framework. Many popular applications — 3ds MAX, Maya, GIMP among others — support Python for automating the application or for creating plugins.
Hello World
print(”Hello, World”)
It’s hard to make things any simpler than this, and Python is often about making things as simple as they can be.
Sort
def insertion_sort(
ulist
):
for idx
in range(1,
len
(
ulist
)):
curr_value = ulist[idx]
pos = idx
while pos > 0 and ulist[pos - 1] > curr_value:
ulist[pos] = ulist[pos - 1]
pos -= 1
ulist[pos] = curr_value
Python code tends to be self-documenting with the tendency of many Python programmers to use longer variable and function names in Snake Case rather than CamelCase.
Of course, the above code need not be written in Python since it comes with an inbuilt function called sort. Python’s sort happens to implement an excellent sorting algorithm that was invented for Python itself. It is called Timsort after its inventor Tim Peters and is widely used .
Tools and Learning Resources
You would be forgiven for believing that a major part of the internet was actually composed of Python tutorials. Even so, Mark Pilgim’s “Dive into Python” and “Dive into Python 3” books are great introductory texts. Another great book is “Think Python: How to Think Like a Computer Scientist”. It too is available for free here. The folks at interactive python have also converted the above book, among others, into an interactive online version that lets you run code samples and run your own code right in the browser. Browser-based tools for Python are now abundant, thanks to Skulpt, a JavaScript ‘port’ of the Python interpreter. You can run basic Python code online here and here. There are also some specialised Python-based online tools available. Morph.io lets you write Python scrapers for extracting data from other websites; PythonAnywhere focuses on hosting Python code and Wakari lets you do scientific computing using Python.
You should definitely prefer the interactive version of the book, unless you have an excessively slow computer or internet connection.
Speaking of scientific computing, packages like NumPy allow one to perform extensive mathematical operations, SymPy allows for symbolic mathematics, Pandas is great for data manipulations, and matplotlib is brilliant for plotting data.
One of the greatest tools though is probably IPython, an enhanced Python shell that integrates well with the above to let you show plots embedded in your Python console. IPython notebook lets you create interactive documents that contain a mixture of rich text, plots and calculations.
There are even specialised versions of Python that come with these packages pre-installed, such as Enthought’s Canopy, Continuum Analytics’ Anaconda, and Python(x,y).
The Viz Mode in online Python IDE CodeSkulptor lets you visualise your Python code as it runs.
Speaking of specialised versions, it’s important to note that the Python installers you get on python.org are just one implementation of the Python language. The three implementations mentioned above bundle tonnes of scientific tools with Python, then there is eGenix Python that’s a single exe! There are also complete re-implementations of Python. For instance JPython is a Python interpreter that runs on the Java VM, and as such it can access Java functionality. Similarly, you have IronPython that runs on .NET Most interesting is PyPy, a Python implementation that was originally written in Python itself. Now it’s a JIT compiler — the kind you find for JavaScript in modern browsers — for Python. In many cases it performs significantly faster than the standard Python implementation. We would never forgive ourselves if we didn’t mention virtualenv, a very popular package for Python that makes developing apps much simpler. It allows you to create virtual Python environments which lets you develop and test your code in a clean environment that isn’t tainted by all the Python packages you have installed globally.
For tutorials on 15 other hot programming languages go here.