Android app development: Intents & more
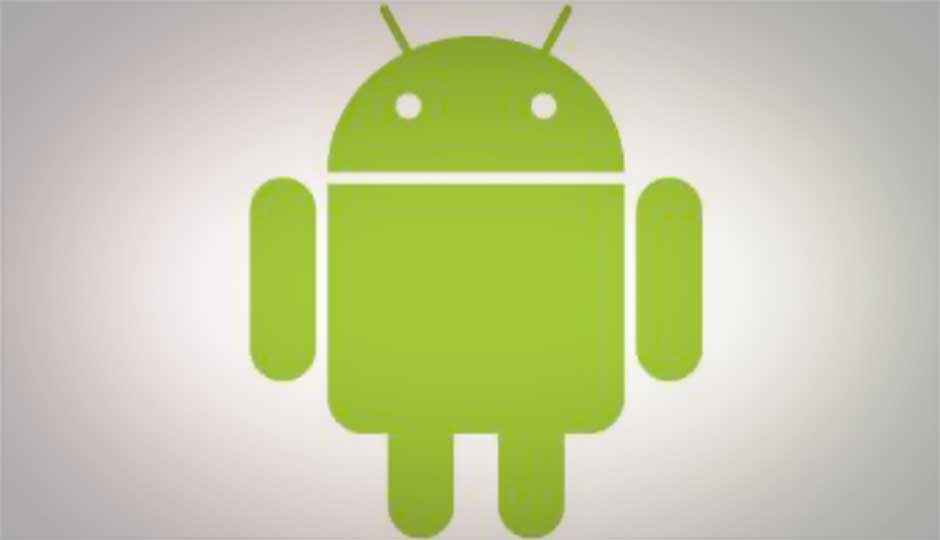
Going further in our journey towards building android applications, we will be modifying the application already created in our previous article. As opposed to earlier, instead of showing the greeting message in an alert dialog we will be showing it in a new activity with the help of a TextView component. This greeting in the second activity will depend on our selection of the festival made in the first activity and will be displayed when the “Show Greeting” button is pressed. This creates a problem, i.e. how do we make the two activities communicate? Moreover, how do we invoke the second activity? This is done with the help of intents. Intents are a mechanism for inter process communication in Android, and this is what we are going to learn.
.jpg)
Intents are objects that carry the identification information for the destined activity, along with some extra data to be communicated to that activity. To communicate with another activity, we just create an object of the Intent class, add information to it and then pass it to methods like startActivity() etc. The receiver need not write any code in order to receive the intent itself. However, in order to access the information stored in the intent object, the object of the calling intent of the current activity can be accessed with the help of getIntent() method of the Activity class.
The extra information stored in the incoming intent is retrieved with the help of its key name in the getStringExtra() method which returns a value of string type. In this code snippet, which will be used in the GreetingReceiver activity for our example, we are checking the vlue of the festival selected by the user and accordingly setting the name of the greeting in the TextView of that activity. Note that this new activity that we create needs to have its own layout file to specify in XML. Let’s name it receiver.xml and create it under res/layout in the folder structure. Thus, the new layout will be accessible with the help of the following line of code :
According to their method of resolution, intents can be explicit or implicit. We will try out small examples of each of these. Explicit Intents Intents which are explicit, specify the name of the Activity which has to receive the intent. Explicit intents are usually used within a specific application, when the destination activity is tailored to receive that intent and information about its existence is known beforehand. In the previous example we called the showDialog() method and overrided the onCreateDialog() method of Activity class to populate the components. Here we will write our own method called launch() that does all the tasks of creating the intent and passing it to the startActivity() method to launch another activity. Basically, this method does nothing but takes the id of the festival passed by the onClickListener and then adds extra information about the festival selected by the radio button. This method should look like as follows:
Now, all we need to do is call this method with the appropriate id as the parameter. The name of the intent receiving activity’s class i.e. GreetingReceiver.class is passed as a parameter to the constructor along with the current package context. This will ensure that this particular activity receives the intent. The putExtra() method takes a set of key-value pairs that are added to the intent and can be extracted from the receiving activity. These are like the extra payload on the android object that is passed along with other information. Finally, we have passed the intent object into the startActivity method. It is useful to note that the receiving activity in this case need not have any configuration or code written to receive such an intent. Implicit Intents Implicit intents need a detailed understanding of how the android framework actually works inside. In such type of intents, we specify what is called an intent filter. This intent filter carries information about what kind of intents a component can receive. If the incoming intent passes through all the criteria specified by this filter, only then can the underlying component react to it, be it broadcast receiver, activity or a service. We can configure an activity to receive an implicit intent in the AndroidManifest.xml file. This can be done as follows:
We can see that the intent filter specifies the action and category for the incoming intent to be received. Actually we can specify an action, category or data. Each of these is matched with those of the intent received and if more than one components capable of receiving an intent are found, the android framework asks the user about what application they want to use. You can take a look at the android documentation if you are more interested in Implicit Intents. In our case, we will implement a simple example that sets a android.content.Intent.ACTION_VIEW property for the action in the intent object. By default, this intent is created with a default category. If you select the Greeting v2 option you will be able to see the greeting according the option selected by the user.
We created two simple examples for us that show how to implement message passing in android and communication between two activities with the help of explicit and implicit intents. The complete source code for each of these applications, each for Explicit and Implicit intents can be accessed here and here.